[Python][華為云Python編程創造營][課堂實驗代碼][進階篇]
Python中的"公式"
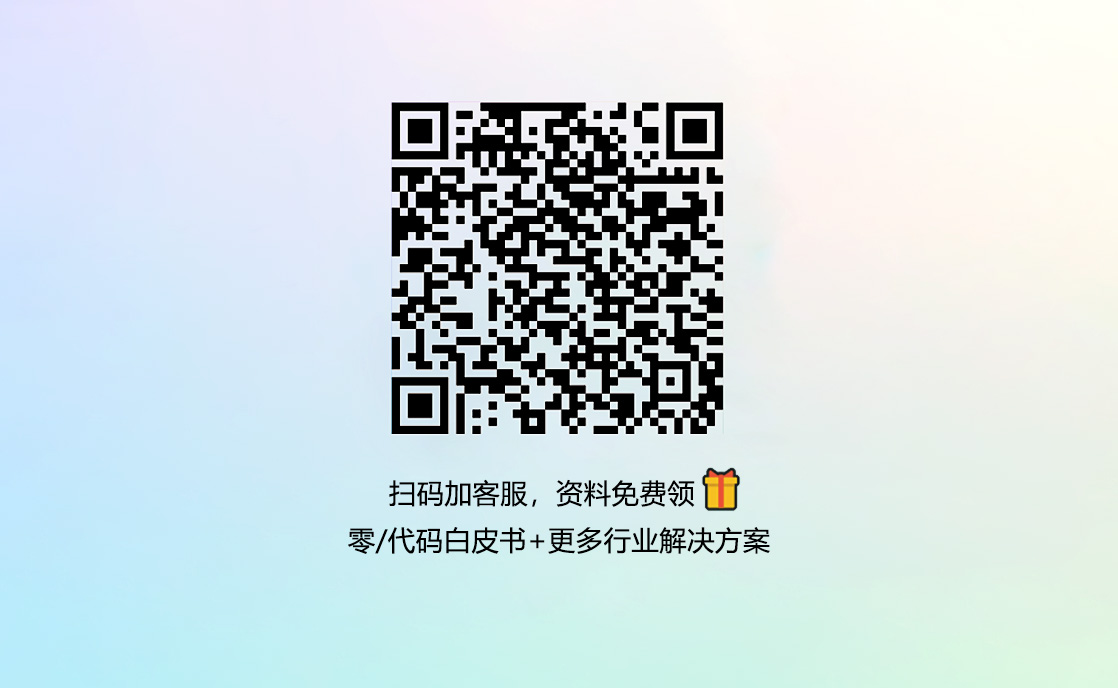
實驗介紹
實驗介紹了對于Python中函數組成、定義及使用,以及Python高階函數的使用
實驗代碼
步驟1,新建名稱為Python函數的使用文件
步驟2,使用def實現Python中的自定義函數
''' 使用函數的形式實現如下功能:返回一個序列(長度為10),序列中每個數字都是之前兩個數字的和 (斐波那契數列,前兩位是0和1) ''' def fibonaccis(): result = [0, 1] # 新建列表存儲數列的值 for i in range(2, 10): # 循環num-2次 a = result[i - 1] + result[i - 2] result.append(a) # 將值追加至列表 return result print(fibonaccis()) # [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
步驟3,函數中返回值的使用
''' 定義函數,實現輸出一個正方形,不添加返回值 ''' # def square(): # print(''' # ------ # | | # ------ # ''') # a=square() # print(a) ''' 定義函數,在函數內部定義多個變量,并且全部返回 ''' # def func(): # a='a' # b='b' # c='c' # return a,b,c # print(func()) #('a', 'b', 'c') ''' 定義函數,在函數內部再次定義一個函數,將內部函數作為返回值返回 ''' # def func1(): # def func2(): # print('內函數調用') # return func2 # b=func1() # print(b) #
步驟4,在函數中使用參數
''' 定義函數,用于計算兩數之和,參與計算的數字使用參數的形式傳遞給函數 ''' def add(a, b, c): return a + b + c print(add(1, 2, 3)) # 6 ''' 在函數中使用位置參數 ''' print(add(a=5, c=1, b=8)) # 14 ''' 使用默認參數 ''' def add(a=4, b=9, c=1): return a + b + c print(add()) # 14 print(add(1, 2)) # 4 print(add(c=3)) # 16 ''' 定義函數實現對于接收的數據求和(使用不定長函數) ''' def add(*args): print(args) # (1, 2, 3, 4, 5, 6) return sum(args) print(add(1, 2, 3, 4, 5, 6)) # 21 ''' 字典形式的不定長參數 ''' def add(**args): print(args) # {'a': 4, 'b': 5, 'c': 6} return sum(args.values()) print(add(a=4, b=5, c=6)) # 15 ''' 使用多種參數,參數的位置順序 ''' def add(a, b=1, *args, **kwargs): print(args) # (1, 2, 3, 4) print(kwargs) # {'c': 4, 'd': 6} add(1, 0, 1, 2, 3, 4, c=4, d=6)
步驟5,函數的說明文檔
def add(a, b): ''' 用于計算兩個數字之和 :param a: 數值類型 :param b: 數值類型 :return: 兩個數字之和 ''' return a + b help(add) ''' Help on function add in module __main__: add(a, b) 用于計算兩個數字之和 :param a: 數值類型 :param b: 數值類型 :return: 兩個數字之和 '''
步驟6,在函數中使用全局變量
a = 10 def func(): print(a) func() #10 ''' 修改全局變量 ''' a = 10 def func(): print(a) a += 1 return a func() #UnboundLocalError: local variable 'a' referenced before assignment
步驟7,全局變量和局部變量重名
# name='吳彥祖' # def func(): # name='小吳' # print('有人喊你,%s'%(name)) # func() #有人喊你,小吳 ''' 使用global關鍵字在函數中聲明這是一個全局變量 ''' # name='吳彥祖' # def func1(): # global name # print('我就是,%s'%(name)) #我就是,吳彥祖 # name='小吳' # print('有人喊你,%s'%(name)) #有人喊你,小吳 # func1() ''' 在函數中修改全局變量 ''' a = 10 def func(): global a a += 1 return a func() print(a) # 11
步驟8,可變類型的數據作為參數對于函數的影響
''' 在函數中使用可變數據類型作為參數時,即使不使用return和global,也會影響結果 ''' list1 = [1, 2, 3] def func(): list1.append(4) func() print(list1) #[1, 2, 3, 4]
步驟9,匿名函數
''' 使用lambda定義匿名函數,實現兩個數相加 ''' y = lambda x1, x2: x1 + x2 print(y(1, 2)) #3
步驟10,函數的執行順序
print(1) def func(): print(2) print(3) func() ''' 輸出結果: 1 3 2 '''
步驟11,def和lambda的嵌套使用
def func(): temp = [lambda x: x * i for i in range(4)] return temp for m in func(): print(m(3)) # 9 9 9 9 ''' 思考:為什么輸出結果是這樣? 答案就在官方文檔里面https://docs.python.org/3.9/library/stdtypes.html#range range(stop) -> range object range(start, stop[, step]) -> range object Return an object that produces a sequence of integers from start (inclusive) to stop (exclusive) by step. range(i, j) produces i, i+1, i+2, ..., j-1. start defaults to 0, and stop is omitted! range(4) produces 0, 1, 2, 3. These are exactly the valid indices for a list of 4 elements. When step is given, it specifies the increment (or decrement). '''
步驟12,高階函數的使用
def add(x, y, f): return f(x) + f(y) # print(add(-5,6,abs())) #TypeError: abs() takes exactly one argument (0 given) print(add(-5, 6, abs)) # 11
步驟13,Python中內置的強大函數
''' zip函數,同時遍歷多個序列 ''' a = [1, 2, 3, 4] b = 'ABCDE' c = ('a', 'b', 'c',) for i in zip(a, b, c): print(i, end=' ') #(1, 'A', 'a') (2, 'B', 'b') (3, 'C', 'c') ''' sorted,對序列進行排序 ''' list1 = [99, -101, 15, 0] print('排序:', sorted(list1)) #排序: [-101, 0, 15, 99] print('按照絕對值排序:', sorted(list1, key=abs)) #按照絕對值排序: [0, 15, 99, -101] print('排序后源數據:', list1) #排序后源數據: [99, -101, 15, 0] ''' filter,過濾序列 ''' list2 = [99, -100, 15, 0] print(list(filter(lambda x: x % 2 == 0, list2))) #[-100, 0] ''' map,序列映射 ''' f = lambda x: x ** 2 + +1 left = [1, 2, 3, 4, 5] right = map(f, left) print(list(right)) #[2, 5, 10, 17, 26] ''' reduce,累計計算 ''' from functools import reduce def fn(x, y): return x * 10 + y print(reduce(fn, [1, 3, 5, 7, 9])) #13579
步驟14,遞歸
''' 使用遞歸實現快速排序算法 ''' def quick_sort(arr): if len(arr) < 2: return arr # 選取基準,隨便選哪個都可以,選中間的便于理解 mid = arr[len(arr) // 2] # 定義基準值左右兩個數列 left, right = [], [] # 從原始數組中移除基準值 arr.remove(mid) for item in arr: # 大于基準值放右邊 if item >= mid: right.append(item) else: # 小于基準值放左邊 left.append(item) # 使用迭代進行比較 return quick_sort(left) + [mid] + quick_sort(right) print(quick_sort([1, 10, 9, 5, 8, 0])) # [0, 1, 5, 8, 9, 10] ''' 一行代碼實現快速排序 ''' quick_sort1 = lambda array: array if len(array) <= 1 else quick_sort1([item for item in array[1:] if item <= array[0]]) + [array[0]] + quick_sort1([item for item in array[1:] if item > array[0]]) print(quick_sort1([1, 10, 9, 5, 8, 0])) # [0, 1, 5, 8, 9, 10]
讓Python給你一個對象
實驗介紹
實驗介紹了對于Python中類和對象的定義及使用,以及Python中魔法方法、設計模式的使用。
實驗代碼
Python中的面向對象
''' 創建Dog類,根據Dog類創建的每個實例都將存儲名字和年齡。我們將賦予每條小狗蹲下(sit())和打滾(roll_over())的能力 ''' class Dog(): '''一次模擬小狗的簡單嘗試''' def __init__(self, name, age): '''初始化屬性name和age''' self.name = name self.age = age def sit(self): '''模擬小狗被命令時蹲下''' print(self.name.title() + " is now sitting") def roll_over(self): '''模擬小狗被命令時打滾''' print(self.name.title() + " rolled over!") # 對類進行實例化 dog = Dog('哈士奇', 2) dog.sit() #哈士奇 is now sitting dog.roll_over() #哈士奇 rolled over!
class Employee(object): '所有員工的基類' empCount = 0 def __init__(self, name, salary): self.name = name self.salary = salary Employee.empCount += 1 def displayCount(self): print('Total Employee %d' % Employee.empCount) def displayEmployee(self): print('Name: ', self.name, ', Salary: ', self.salary) # 創建Employee類的第一個對象 emp1 = Employee('Zara', 100) # 創建Employee類的第二個對象 emp2 = Employee('Manni', 100) emp1.displayEmployee() emp2.displayEmployee() print('Total Employee %d' % Employee.empCount)
''' 在創建類并實例化對象后,動態的修改類 ''' class Dog(): def __init__(self, name, age): '''初始化屬性name和age''' self.name = name self.age = age dog = Dog('大寶', 0.5) Dog.color = 'yellow' print(dog.color) #yellow ''' 為類添加方法 ''' def eat(self): print('%s吃東西' % self.name) Dog.eat = eat dog.eat() #大寶吃東西 ''' 在對類添加屬性和方法時,對應的實例化的對象也會收到影響 ''' # 為實例化的對象添加屬性和方法 dog.weight = 10 def bark(): print('叫。。。') dog.bark = bark print(dog.weight) #10 print(dog.bark()) #叫。。。 dog2 = Dog('旺財', 1) print(dog2.weight) #AttributeError: 'Dog' object has no attribute 'weight' print(dog2.bark()) #AttributeError: 'Dog' object has no attribute 'bark'
# 定義父類 class Parent(object): parentAttr = 100 def __init__(self): print('調用父類構造函數') def parentMethod(self): print('調用父類方法') def setAttr(self, attr): Parent.parentAttr = attr def getAttr(self): print('父類屬性:', Parent.parentAttr) # 定義子類 class Child(Parent): def __init__(self): print('調用子類構造函數') def childMethod(self): print('調用子類方法') c = Child() #實例化子類 c.childMethod() #調用子類的方法 c.parentMethod() #調用父類的方法 c.setAttr(200) #再次調用父類的方法 - 設置屬性值 c.getAttr() #再次調用父類的方法 - 獲取屬性值 ''' 輸出結果: 調用子類構造函數 調用子類方法 調用父類方法 父類屬性: 200 '''
''' 創建父類Dog,具備屬性年齡和姓名,會吃飯,會叫 ''' class Dog(object): def __init__(self, name, age): self.name = name self.age = age def eat(self, food): print('%s吃%s' % (self.name, food)) def bark(self): print('汪汪汪') ''' 創建父類Wolf,具備屬性姓名、顏色,會捕獵,會叫 ''' class Wolf(object): def __init__(self, name, color): self.name = name self.color = color def bark(self): print('嗚嗚嗚') def hunt(self, prey): print('%s狩獵了%s' % (self.name, prey)) ''' 多繼承實現新的類Husky ''' class Husky(Dog, Wolf): def destory(self, master): if not master: print('哈士奇開始搞破壞啦') husky = Husky(age='3', name='二哈') husky.hunt('沙發') #二哈狩獵了沙發 husky.eat('沙發') #二哈吃沙發 husky.bark() #汪汪汪 husky.destory(0) #哈士奇開始搞破壞啦 # 當繼承多個類時,優先繼承排在前面的類(同名屬性和方法)
class Person(object): def __init__(self, name, age): self.name = name self.age = age self.__friend = ['lisi', 'wangwu'] def __play(self): print('打籃球') class child(Person): pass c = child('zhangsan', 18) # print(c.friend) #AttributeError: 'child' object has no attribute 'friend' c.__play() #AttributeError: 'child' object has no attribute '__play'
''' 通過字符串的形式開判斷當前對象是否存在該屬性或者方法 hasattr(obj,name_str):判斷object是否有name_str這個方法或者屬性 getattr(obj,name_str):獲取object對象中與name_str同名的方法或者函數 setattr(obj,name_str,value):為object對象設置一個以name_str為名的value方法或者屬性 delattr(obj,name_str):刪除object對象中的name_str方法或者屬性 ''' class Dog(object): def __init__(self, age, name): self.age = age self.name = name def eat(self, food): print('%s吃%s' % (self.name, food)) def bark(self): print('汪汪汪') dog = Dog(3, '大寶') if hasattr(dog, 'eat'): eat = getattr(dog, 'eat') food = input('你要喂它吃什么:') eat(food) setattr(dog, 'temp', '飽') print('大寶吃%s了' % dog.temp) ''' 輸出結果: 你要喂它吃什么:- 大寶吃- 大寶吃飽了 '''
isinstance(dog, Dog) # True
class P(object): num = 0 def __init__(self): P.num += 1 @classmethod def get_no_of_instance(cls): return cls.num @staticmethod def func(): return '這是一個靜態方法' p1 = P() p2 = P() print(P.get_no_of_instance()) #2 print(P.func()) #這是一個靜態方法 print(p1.func()) #這是一個靜態方法
魔法方法的使用
# 在同一個類中使用這兩個魔法方法 class person(): def __init__(self): print('調用init') def __new__(cls, *args, **kwargs): print('調用new') return object.__new__(cls, *args, **kwargs) p = person() ''' 輸出結果: 調用new 調用init '''
# 在對象刪除時,調用的方法 class person(): def __del__(self): print('這個對象被刪除了') p = person() del (p) #這個對象被刪除了
class obj(object): def __init__(self, value): self.value = value def __len__(self): return len(self.value) - 1 o = obj([1, 2, 3]) print(len(o)) #2
# 定義對象時,不重構__str__和__repr__ class obj(object): pass o = obj() print(o) #<__main__.obj object at 0x0000020A41BE8FD0> print(str(o)) #<__main__.obj object at 0x0000020A41BE8FD0> print(repr(o)) #<__main__.obj object at 0x0000020A41BE8FD0> class obj1(object): def __str__(self): return '這個obj1實例化的一個對象' def __repr__(self): return '調用了repr' o = obj1() print(o) #這個obj1實例化的一個對象 print(str(o)) #這個obj1實例化的一個對象 print(repr(o)) #調用了repr
Python中類的屬性的操作
class People(object): def __init__(self, name, age): self.name = name self.age = age def __getattribute__(self, obj): #重寫__getattribute__方法,形參obj是訪問的屬性,是一個屬性名字字符串 if obj == 'age': print('問別人年齡是不禮貌的') return 18 else: #else此處為其他訪問屬性訪問過程,如果不寫,那age和show方法就訪問不到了 temp = object.__getattribute__(self, obj) return temp #不寫return的話,show方法無法執行 def show(self): print('---') s = People('python', 30) print(s.age) print(s.name) s.show() ''' 輸出結果: 問別人年齡是不禮貌的 18 python --- '''
# 讓方法像屬性一樣調用 class Dog(object): def __init__(self, age, name): self.age = age self.name = name @property def bark(self): print('汪汪汪') dog = Dog(1, 'BayMAX') dog.bark #汪汪汪
''' 獲取私有屬性并賦值 ''' class Dog(object): def __init__(self, age, name): self.age = age self.name = name @property def age(self): return self.__age @age.setter #設置屬性賦值要求 def age(self, Age): if 0 < Age < 100: self.__age = Age return self.__age else: self.__age = 18 return self.__age dog = Dog(1, 'BayMAX') dog.age = 10 print(dog.age) #10 dog.age = 130 print(dog.age) #18 ''' 在類中設置只讀屬性 ''' class Dog(object): def __init__(self, age, name): self.__age = age self.name = name @property def age(self): return self.__age @age.getter def age(self): return self.__age dog = Dog(1, 'BayMAX') print(dog.age) #1 dog.age = 10 #AttributeError: can't set attribute # 不設置setter對應的方法即為只讀
設計模式基礎
# 實現手機類,通過工廠來生產不同的手機 class Phone(object): def __init__(self, color, price): self.color = color self.price = price def get_price(self): return self.price class DaMi(Phone): def __init__(self, name): print('這是一臺:%s' % name) class XiaMi(Phone): def __init__(self, name): print('這是一臺:%s' % name) class Factory(object): def getPhone(self, name, brand): if brand == 'DaMi': return DaMi(name) if brand == 'XiaMi': return XiaMi(name) factory = Factory() phone = factory.getPhone('大米11', 'DaMi') #這是一臺:大米11
# 實例化一個單例 class Singlton(object): __instance = None def __new__(cls, age, name): # 如果類屬性__instance的值為None # 那么就創建一個對象,并且賦值為這個對象的引用,保證下次調用這個方法時 # 能夠知道之前已經創建過對象了,這樣就保證了只有1個對象 if not cls.__instance: cls.__instance = object.__new__(cls) return cls.__instance a = Singlton(18, '張三') b = Singlton(8, '李四')
# 此處通過Python中的裝飾器實現單例模式(了解) def singleton(cls): # 單下劃線的作用是這個變量只能在當前模塊里訪問,僅僅是一種提示作用 # 創建一個字典用來保存類的實例對象 _instance = {} def _singleton(*args, **kwargs): # 先判斷這個類有沒有對象 if cls not in _instance: _instance[cls] = cls(*args, **kwargs) #創建一個對象,并保存到字典當中 # 將實例對象返回 return _instance[cls] return _instance @singleton class A(object): a = 1 def __init__(self, x=0): self.x = x print('這是A的類的初始化方法') a1 = A(2) a2 = A(3) a1 is a2
“發明輪子"和"造輪子”
實驗介紹
實驗介紹了對于Python中常用標準模塊的使用
實驗代碼
步驟1,導入模塊
''' # 新建一個Python文件a.py ''' def funca(): print('funca被調用') A = '我來自funca' def funcb(): print('funcb被調用') # 調用寫好的a.py import a a.funca() #funca被調用 print(a.A) #我來自funca from a import funcb funcb() #funcb被調用 from a import * funca() #funca被調用 import a as AAA AAA.funca() #funca被調用
步驟2,os模塊常用函數的使用
import os # os.getpid() 獲取當前進程id print('當前進程的ID:', os.getpid()) # os.getppid() 獲取當前父進程id print('當前父進程的ID:', os.getppid()) # os.getcwd() 獲取當前所在路徑 cwd = os.getcwd() print('當前所在路徑為:', cwd) # os.chdir(path) 改變當前工作目錄 os.chdir('C:\\') print('修改后當前所在路徑為:', os.getcwd()) # os.listdir() 返回目錄下所有文件 print('當前目錄下的文件有:', os.listdir(cwd)) # os.walk() 輸出當前路徑下的所有文件 for root, dirs, files in os.walk(cwd, topdown=False): for name in files: print(os.path.join(root, name)) for name in dirs: print(os.path.join(root, name))
步驟3,os文件操作
import os # 獲取當前工作目錄的名稱 os.curdir # 父目錄字符串名稱 os.pardir # 刪除指定文件 os.remove() # 刪除文件夾 os.removedirs(path) # 重命名文件 os.rename(src, dst) # 命名前,命名后
步驟4,os path子模塊
import os # os.path.abspath(path):返回絕對路徑 print('text.txt的絕對路徑為:', os.path.abspath('text.txt')) #text.txt為當前文件夾下的一個文件 # os.path.exists(path):文件存在則返回True,不存在返回False print('text.txt是否存在:', os.path.exists('text.txt')) # os.path.getsize(path):返回文件大小,如果文件不存在就返回錯誤 print('text.txt的文件大小:', os.path.getsize('text.txt')) # os.path.isfile(path):判斷路徑是否為文件 print('text.txt是否為文件:', os.path.isfile('text.txt')) # os.path.isdir(path):判斷路徑是否為文件夾 print('text.txt是否為文件夾:', os.path.isdir('text.txt'))
步驟5,sys模塊的使用
''' sys.exit([n]) 此方法可以是當前程序退出,n為0時表示正常退出,其它值表示異常退出 ''' import sys for i in range(100): print(i) if i == 5: sys.exit(0) ''' 輸出結果 0 1 2 3 4 5 ''' ''' sys.path 獲取模塊搜索路徑 ''' sys.path ''' sys.argv 從程序外部向程序傳參數,參數以列表形式傳遞,第一個為當前文件名 ''' # 新建test.py文件(在當前文件夾下或者桌面),寫入以下代碼 print(sys.argv[1]) # 在命令行中切換到文件路徑,運行程序 # python text.py hello # 獲取Python解釋器版本 sys.version # 獲取默認編碼格式 sys.getdefaultencoding()
步驟6,time模塊的使用
from logging import logThreads import time # time.time() 用于獲取當前時間戳 time_now = time.time() print('時間戳:', time_now) #時間戳: 1646606123.3409111 # time.localtime() 獲取時間元組 localtime = time.localtime(time_now) print('本地時間為:', localtime) #本地時間為: time.struct_time(tm_year=2022, tm_mon=3, tm_mday=7, tm_hour=6, tm_min=39, tm_sec=21, tm_wday=0, tm_yday=66, tm_isdst=0) # time.asctime() 獲取格式化時間 localtime = time.asctime(localtime) print('本地時間為:', localtime) #本地時間為: Mon Mar 7 06:40:08 2022 # time.strftime(format[,t]) 接收時間元組,并返回以可讀字符串表示的當地時間,格式由參數format決定 print(time.strftime('%Y-%m-%d %H:%M:%S', time.localtime())) #2022-03-07 06:41:44
步驟7,random模塊的使用
import random print(random.randint(1, 10)) # 產生1到10的一個整數型隨機數 print(random.random()) # 產生0到1之間的隨機浮點數 print(random.uniform(1.1, 5.4)) # 產生1.1到5.4之間的隨機浮點數,區間可以不是 ''' 整數 ''' print(random.choice('tomorrow')) # 從序列中隨機選取一個元素 print(random.randrange(1, 100, 2)) # 生成1到100的間隔為2的隨機整數 print(random.sample('python', 3)) # 取3個值 list1 = [1, 2, 3, 4] random.shuffle(list1) # 洗牌,打亂序列 print(list1)
Python也要你的文件讀寫權限
實驗介紹
本實驗介紹了Python open函數的使用和Python讀取常見格式的數據文件
實驗代碼
Python文件讀寫
''' 使用Python內建的open函數打開一個文本文件(本地已經存在的) ''' f = open('text.txt', 'r', encoding='utf8') print(f.read()) f.close() ''' 輸出結果: hello world text.txt ''' ''' 文件太大時,只讀取一部分 ''' f = open('text.txt', 'r', encoding='utf8') print(f.read(1)) #h f.close() ''' 打開一個不存在的文件(使用只讀模式) ''' f = open('t.txt', 'r', encoding='utf8') f.read() #FileNotFoundError: [Errno 2] No such file or directory: 't.txt'
''' 使用不同模式寫入文本到文件中,使用w模式 ''' str_ = ''' 一二三四五 六七八九十 ''' f = open('text.txt', 'w', encoding='utf8') f.write(str_) f.close() ''' 文本內容: 一二三四五 六七八九十 ''' ''' 使用a模式寫入 ''' str_ = ''' 射雕英雄傳/金庸 ''' f = open('text.txt', 'a', encoding='utf8') f.write(str_) f.close ''' 文本內容: 一二三四五 六七八九十 射雕英雄傳/金庸 ''' ''' 使用r模式嘗試寫入 ''' str_ = ''' --射雕英雄傳/金庸 ''' f = open('text.txt', 'r', encoding='utf8') f.write(str_) #io.UnsupportedOperation: not writable f.close()
''' 讀取一行 ''' f = open('text.txt', 'r', encoding='utf8') line = f.readline() lines = f.readlines() print(line) print(lines) # ['一二三四五\n', '六七八九十\n', '\n', '射雕英雄傳/金庸\n']
str_ = ''' --射雕英雄傳/金庸 ''' f = open('text_new.txt', 'a', encoding='utf8') f.write(str_) # 此處不使用close關閉文件 ''' 讀取這個沒保存的文件 ''' f_new = open('text_new.txt', 'r', encoding='utf8') f_new.read() # 輸出為空
str_ = ''' 射雕英雄傳/金庸 ''' f = open('text_new.txt', 'a', encoding='utf8') f.write(str_) f.flush() ''' 不保存,直接讀取 ''' f_new = open('text_new.txt', 'r', encoding='utf8') print(f_new.read()) ''' 輸出內容 射雕英雄傳/金庸 射雕英雄傳/金庸 ''' # 在flush方法中一并寫入到文件中(模式為a)
# tell f = open('text.txt', 'r', encoding='utf8') a = f.read(10) print(f.tell()) #28
# 使用上下文管理器打開文件 with open('text.txt', 'a', encoding='utf8') as f: f.write('這本小說很好看') with open('text.txt', 'r', encoding='utf8') as f: print(f.read()) ''' 輸出內容 一二三四五 六七八九十 射雕英雄傳/金庸 這本小說很好看 '''
Python讀取其他數據文件
''' 使用open函數讀取 ''' with open('huawei_logo.png', 'r', encoding='utf8') as f: print(f.read()) ''' 使用二進制形式打開 ''' with open('huawei_logo.png', 'rb') as f: img = f.read() print(type(img)) print(img) # bytes類型是指一堆字節的集合,在Python中以b開頭的字符串都是bytes類型 ''' 獲取圖片的像素矩陣 ''' import cv2 # 此處使用opencv讀取,可以換其他工具 img = cv2.imread('huawei_logo.png') print(img)
''' 使用open函數讀取 ''' with open('music.wav', 'rb') as f: print(f.read()) ''' 獲取音頻的矩陣表示 ''' from scipy.io import wavfile wav_data = wavfile.read('music.wav') wav_data
import pandas as pd df = pd.read_csv('air.csv', names=['city', 'pm2.5', 'level'], encoding='utf8') df # 讀取excel文件使用read_excel方法即可,但是需要事先安裝額外的依賴包xlrd
Python
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。