最簡單的 UE 4 C++ 教程 —— 觸發區域內的鍵盤響應開關燈【二十五】
原教程是基于 UE?4.18,我是基于 UE 4.25】
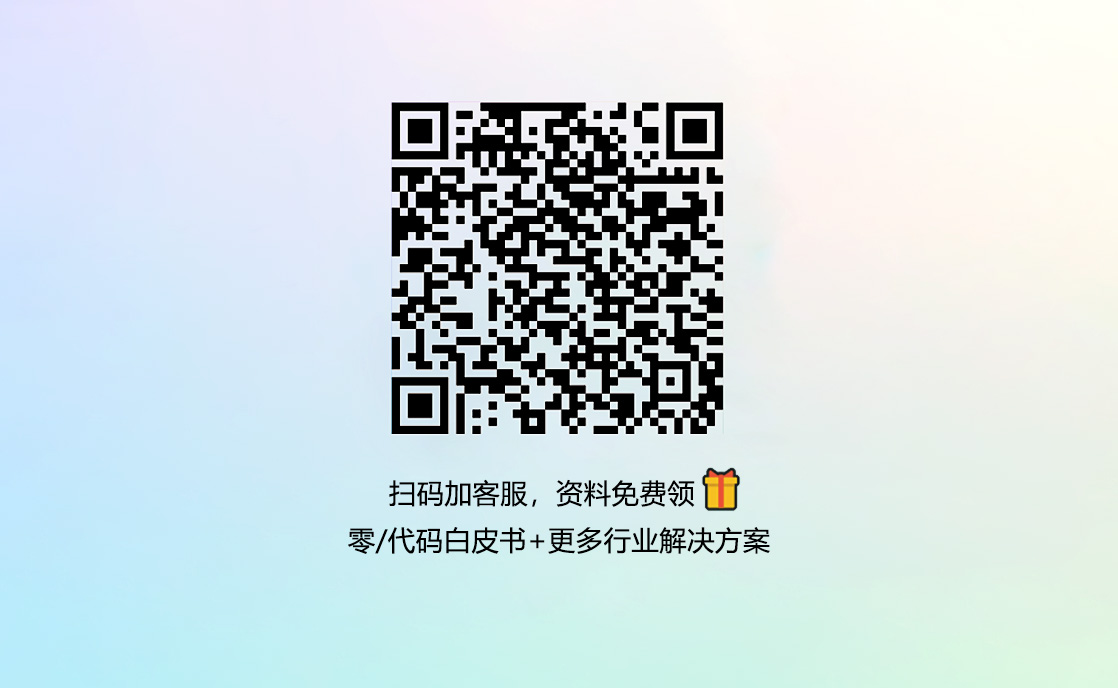
英文原地址
接上一節教程,創建一個新的 C++ Actor 子類并將其命名為 LightSwitchPushButton 。我們將在頭文件中定義四個東西?—— 我們將定義一個 UPointLightComponent、USphereComponent、float 變量和 void 函數。
下面是最終的頭代碼。
LightSwitchPushButton.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "LightSwitchPushButton.generated.h"
UCLASS()
class UNREALCPP_API ALightSwitchPushButton : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
ALightSwitchPushButton();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
// virtual void Tick(float DeltaTime) override;
// declare point light comp
UPROPERTY(VisibleAnywhere, Category = "Light Switch")
class UPointLightComponent* PointLight;
// declare sphere comp
UPROPERTY(VisibleAnywhere, Category = "Light Switch")
class USphereComponent* LightSphere;
// declare light intensity variable
UPROPERTY(VisibleAnywhere, Category = "Light Switch")
float LightIntensity;
// declare ToggleLight function
UFUNCTION(BlueprintCallable, Category = "Light Switch")
void ToggleLight();
};
接下來,在我們的 .cpp 文件中,讓我們首先 #include 我們將在代碼中使用的必要腳本。包括Components / PointLightComponent.h 和 Components/ spherecomcomponent .h?兩個文件。
#include "LightSwitchPushButton.h"
// include these header files
#include "Components/PointLightComponent.h"
#include "Components/SphereComponent.h"
我們將在構造函數中設置 actor 的所有默認屬性。首先讓我們設置我們的 float 變量 LightIntensity 為 3000.0f,它將使光相對于其他對象看起來足夠明亮。接下來,我們將創建我們的UPointLightComponent 并將它設置為我們的 RootComponent 。之后,我們將創建USphereComponent,當我們的玩家在半徑內時,它將作為碰撞球體。然后,我們將創建簡單的ToggleLight() 函數來切換燈光的可見性狀態。稍后我們將從玩家代碼中調用該函數。下面是LightSwitchPushButton 角色的最后一個 .cpp 文件。
LightSwitchPushButton.cpp
#include "LightSwitchPushButton.h"
#include "Components/PointLightComponent.h"
#include "Components/SphereComponent.h"
// Sets default values
ALightSwitchPushButton::ALightSwitchPushButton()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
LightIntensity = 3000.0f;
PointLight = CreateDefaultSubobject
PointLight->Intensity = LightIntensity;
//PointLight->bVisible = true; ///< 過時了
//PointLight->SetVisibleFlag(true);
PointLight->SetVisibility(true);
RootComponent = PointLight;
LightSphere = CreateDefaultSubobject
LightSphere->InitSphereRadius(300.0f);
LightSphere->SetCollisionProfileName(TEXT("Trigger"));
LightSphere->SetCollisionResponseToChannel(ECC_Pawn, ECR_Ignore);
LightSphere->SetupAttachment(RootComponent);
}
// Called when the game starts or when spawned
void ALightSwitchPushButton::BeginPlay()
{
Super::BeginPlay();
}
void ALightSwitchPushButton::ToggleLight()
{
PointLight->ToggleVisibility();
}
接下來,和上一節類似,讓我們向項目添加一個 Action 輸入。在本例中,我們將把 Action 輸入綁定到鍵盤的 F鍵。轉到 編輯>項目設置 (?Edit > Project Settings)。然后選擇 Input 選項。單擊 Action Mappings 旁邊的加號。調用新的輸入 Action 并從下拉菜單中選擇 F 。
【以下我們把目光轉到 xxxCharacter.h / .cpp 上】
在 xxxCharacter.h文件中,在 OnFire 方法下添加 OnAction 方法。
protected:
/** Fires a projectile. */
void OnFire();
// on action
void OnAction();
此外,我們還必須包含 LightSwitchPushButton 頭文件,這樣我們的角色才能訪問它的功能。
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
// include our new LightSwitchPushButton header file
#include "LightSwitchPushButton/LightSwitchPushButton.h"
#include "UnrealCPPCharacter.generated.h"
然后為玩家當前重疊的燈開關聲明一個變量 CurrentLightSwitch 。此外,我們還需要聲明重疊事件,來使得當玩家處于光的球體組件的半徑內時,觸發我們想要運行的函數。
// declare overlap begin function
UFUNCTION()
void OnOverlapBegin(class UPrimitiveComponent* OverlappedComp, class AActor* OtherActor, class UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult);
// declare overlap end function
UFUNCTION()
void OnOverlapEnd(class UPrimitiveComponent* OverlappedComp, class AActor* OtherActor, class UPrimitiveComponent* OtherComp, int32 OtherBodyIndex);
// declare current light switch
class ALightSwitchPushButton* CurrentLightSwitch;
同時還聲明了?UCapsuleComponent?來處理我們的觸發事件
UPROPERTY(VisibleAnywhere, Category = "Trigger Capsule")
class UCapsuleComponent* TriggerCapsule;
在構造函數中添加觸發器膠囊并將其綁定到重疊事件。接著設置變量?CurrentLightSwitch 為NULL。
AUnrealCPPCharacter::AUnrealCPPCharacter()
{
...
// create trigger capsule
TriggerCapsule = CreateDefaultSubobject
TriggerCapsule->InitCapsuleSize(55.f, 96.0f);;
TriggerCapsule->SetCollisionProfileName(TEXT("Trigger"));
TriggerCapsule->SetupAttachment(RootComponent);
// bind trigger events
TriggerCapsule->OnComponentBeginOverlap.AddDynamic(this, &AUnrealCPPCharacter::OnOverlapBegin);
TriggerCapsule->OnComponentEndOverlap.AddDynamic(this, &AUnrealCPPCharacter::OnOverlapEnd);
// set current light switch to null
CurrentLightSwitch = NULL;
}
進一步,將 Action 輸入綁定連接到玩家
void AUnrealCPPCharacter::SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent)
{
...
// Bind action event
PlayerInputComponent->BindAction("Action", IE_Pressed, this, &AUnrealCPPCharacter::OnAction);
}
將 OnAction() 函數添加到玩家腳本中。該函數將檢查 CurrentLightSwitch 是否為 NULL 。如果CurrentLightSwitch 不為 NULL,那么當玩家按下動作鍵 F 時,將切換燈光的可見性(開關燈)。然后,添加重疊函數來設置和取消 CurrentLightSwitch
void AUnrealCPPCharacter::OnAction()
{
if(CurrentLightSwitch)
{
CurrentLightSwitch->ToggleLight();
}
}
// overlap on begin function
void AUnrealCPPCharacter::OnOverlapBegin(class UPrimitiveComponent* OverlappedComp, class AActor* OtherActor, class UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor && (OtherActor != this) && OtherComp && OtherActor->GetClass()->IsChildOf(ALightSwitchPushButton::StaticClass()))
{
CurrentLightSwitch = Cast
}
}
// overlap on end function
void AUnrealCPPCharacter::OnOverlapEnd(class UPrimitiveComponent* OverlappedComp, class AActor* OtherActor, class UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
if (OtherActor && (OtherActor != this) && OtherComp)
{
CurrentLightSwitch = NULL;
}
}
編譯代碼。拖放 actor (LightSwitchPushButton)到場景中,當玩家進入球形觸發區域,點擊 F 鍵開關燈。
最后的效果圖如下
C++
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。