SpringBoot學習筆記(一、HelloSpringBoot)
一、Springboot簡介
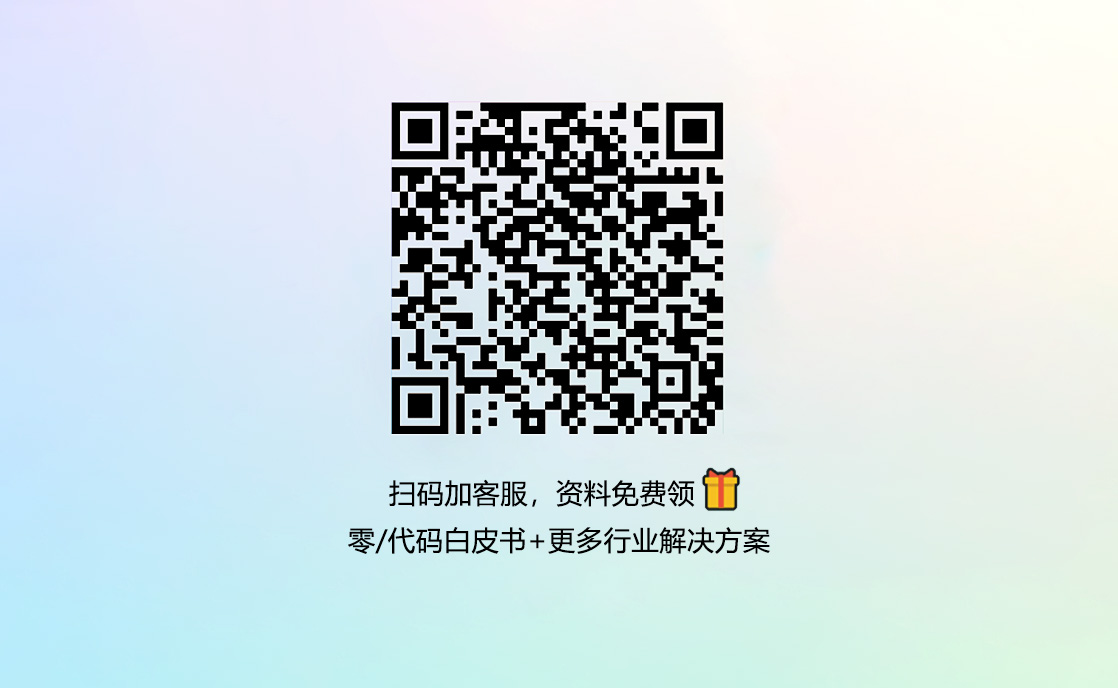
Spring Boot makes it easy to create stand-alone, production-grade Spring based Applications that you can “just run”…Most Spring Boot Applications need very little Spring configuration.
1
這是官方文檔的介紹。
Spring Boot(英文中是“引導”的意思),是用來簡化Spring應用的搭建到開發的過程。應用開箱即用。
在學習SSM(H)的過程中,需要做大量的配置工作,其實很多配置行為本身只是手段,并不是目的。 基于這個考慮,把該簡化的簡化,該省略的省略,開發人員只用關心提供業務功能就行了,這就是 Springboot。
換言之,SpringBoot可以簡單地看成簡化了的、按照約定開發的SSM(H),可以使開發速度大大提升。
二、使用Eclipse創建一個Maven項目
這一步的前提是你的電腦完成了Maven的相關配置。
詳情如圖:
三、具體代碼
1、pom.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
2、 Controller層
HelloController.java
import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class HelloController { @RequestMapping("/hello") public String sayHello() { return "Hello,SpringBoot!"; } }
1
2
3
4
5
6
7
8
9
10
@RestController和@RequestMapping注解是來自SpringMVC的注解,它們不是SpringBoot的特定部分。
(1). @RestController:提供實現了REST API,可以服務JSON,XML或者其他。這里是以String的形式渲染出結果。
(2). @RequestMapping:提供路由信息,”/hello“路徑的HTTP Request都會被映射到sayHello方法進行處理。
3、啟動應用類
Application.java
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; /*Spring Boot啟動類 * */ @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class,args); } }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
四、運行
運行也比較簡單,將Application.java運行一下
好,看到Application運行沒有問題,訪問網址:http://localhost:8080/hello
好的這次啟動沒有問題,到這沒有完,我對Controller作了點修改,刷新網頁,看不到修改內容,那就第二次運行Application.java,結果報了下面這個錯誤。
java.net.BindException: Address already in use: bind at sun.nio.ch.Net.bind0(Native Method) ~[na:1.8.0_144] at sun.nio.ch.Net.bind(Unknown Source) ~[na:1.8.0_144] at sun.nio.ch.Net.bind(Unknown Source) ~[na:1.8.0_144] at sun.nio.ch.ServerSocketChannelImpl.bind(Unknown Source) ~[na:1.8.0_144] at sun.nio.ch.ServerSocketAdaptor.bind(Unknown Source) ~[na:1.8.0_144] at org.apache.tomcat.util.net.NioEndpoint.bind(NioEndpoint.java:340) ~[tomcat-embed-core-8.0.32.jar:8.0.32] at org.apache.tomcat.util.net.AbstractEndpoint.start(AbstractEndpoint.java:765) ~[tomcat-embed-core-8.0.32.jar:8.0.32] at org.apache.coyote.AbstractProtocol.start(AbstractProtocol.java:473) ~[tomcat-embed-core-8.0.32.jar:8.0.32] at org.apache.catalina.connector.Connector.startInternal(Connector.java:986) [tomcat-embed-core-8.0.32.jar:8.0.32] at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:147) [tomcat-embed-core-8.0.32.jar:8.0.32] at org.apache.catalina.core.StandardService.addConnector(StandardService.java:239) [tomcat-embed-core-8.0.32.jar:8.0.32] at org.springframework.boot.context.embedded.tomcat.TomcatEmbeddedServletContainer.addPreviouslyRemovedConnectors(TomcatEmbeddedServletContainer.java:194) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.context.embedded.tomcat.TomcatEmbeddedServletContainer.start(TomcatEmbeddedServletContainer.java:151) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.context.embedded.EmbeddedWebApplicationContext.startEmbeddedServletContainer(EmbeddedWebApplicationContext.java:293) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.context.embedded.EmbeddedWebApplicationContext.finishRefresh(EmbeddedWebApplicationContext.java:141) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:541) [spring-context-4.2.5.RELEASE.jar:4.2.5.RELEASE] at org.springframework.boot.context.embedded.EmbeddedWebApplicationContext.refresh(EmbeddedWebApplicationContext.java:118) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.SpringApplication.refresh(SpringApplication.java:766) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.SpringApplication.createAndRefreshContext(SpringApplication.java:361) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.SpringApplication.run(SpringApplication.java:307) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.SpringApplication.run(SpringApplication.java:1191) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at org.springframework.boot.SpringApplication.run(SpringApplication.java:1180) [spring-boot-1.3.3.RELEASE.jar:1.3.3.RELEASE] at edu.hpu.springboot.Application.main(Application.java:13) [classes/:na]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
這個錯誤的解決辦法查了一下,最后找到一個靠譜的,錯誤原因是8080這個端口被占用,Application雖然跑不起來,http://localhost:8080/hello 照樣能訪問,關于SpringBoot的運行機制我不太清楚,但大概知道之前運行之后應該生成了什么,占用這個端口。所以查一下:
cmd -> netstat -ano
結果:
找到被哪個進程被占用了,打開任務管理器,點擊詳細信息
就是它,javaw.exe,結束任務,再次運行Application.java,訪問http://localhost:8080/hello ,沒有問題,Controller中更改的內容也顯示出來了。
參考:
【1】https://www.w3cschool.cn/springboot/springboot-w7c2245h.html
【2】http://how2j.cn/k/springboot/springboot-eclipse/1640.html
【3】https://www.cnblogs.com/13188196we/p/3278153.html
Java Spring Spring Boot
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。
版權聲明:本文內容由網絡用戶投稿,版權歸原作者所有,本站不擁有其著作權,亦不承擔相應法律責任。如果您發現本站中有涉嫌抄襲或描述失實的內容,請聯系我們jiasou666@gmail.com 處理,核實后本網站將在24小時內刪除侵權內容。