Node.js VM模塊
Executing JavaScript
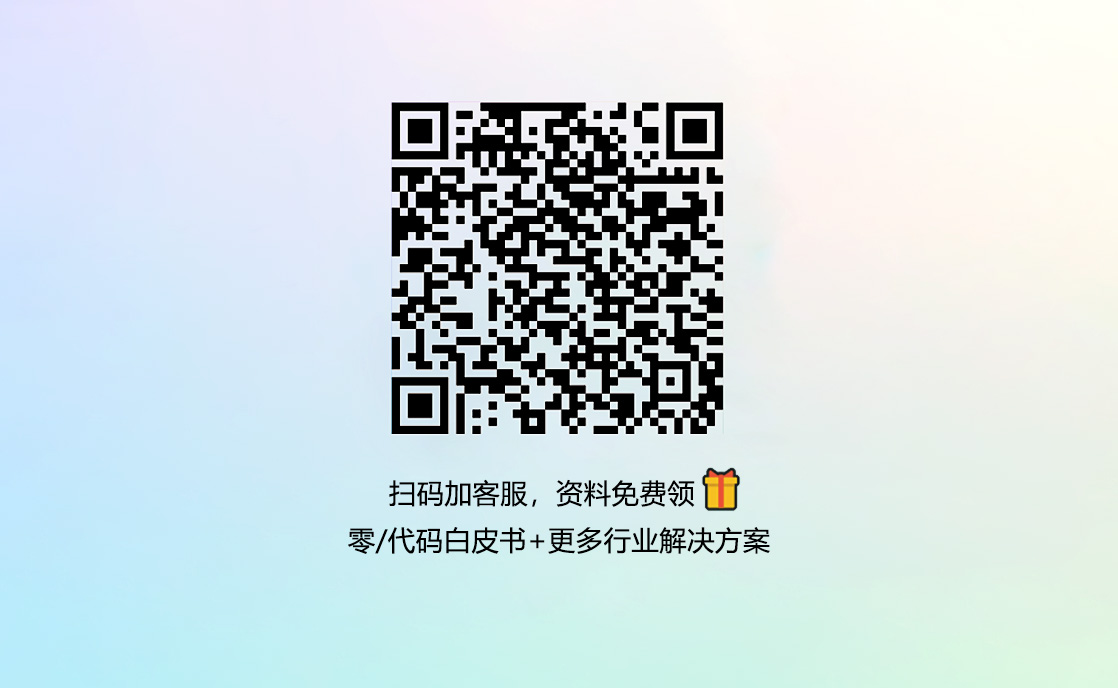
Class: vm.Script
new vm.Script(code, options)
script.runInContext(contextifiedSandbox[, options])
script.runInNewContext([sandbox][, options])
script.runInThisContext([options])
vm.createContext([sandbox])
vm.isContext(sandbox)
vm.runInContext(code, contextifiedSandbox[, options])
vm.runInDebugContext(code)
vm.runInNewContext(code[, sandbox][, options])
vm.runInThisContext(code[, options])
Example: Running an HTTP Server within a VM
What does it mean to “contextify” an object?
Executing JavaScript
執行JavaScript
The vm module provides APIs for compiling and running code within V8 Virtual Machine contexts. It can be accessed using:
vm模塊在V8虛擬機上下文中提供了編譯和運行代碼的API。它可以通過如下方式訪問:
const vm = require('vm')
1
JavaScript code can be compiled and run immediately or compiled, saved, and run later.
JavaScript代碼可以被編譯然后立即運行,或者編譯,保存,并且之后運行。
Class: vm.Script
Added in:
Instances of the vm.Script class contain precompiled scripts that can be executed in specific sandboxes (or “contexts”).
vm.Script類的實例包含的預編譯腳本可以在沙箱中被執行(或者上下文環境中)。
new vm.Script(code, options)
Added in: v0.3.1
code
options
filename
lineOffset
columnOffset
displayErrors
timeout
cachedData
produceCachedData
code
options 選項
filename
lineOffset
columnOffset
displayErrors
timeout
cachedData
produceCachedData
Creating a new vm.Script object compiles code but does not run it. The compiled vm.Script can be run later multiple times. It is important to note that the code is not bound to any global object; rather, it is bound before each run, just for that run.
創建一個新的vm.Script對象編譯代碼但是并不運行。編譯的vm.Script之后可以被多次運行。必須注意的是該代碼并沒有綁定到任何的全局對象;相反的它們只在每次運行前被綁定到那次的運行環境上。
script.runInContext(contextifiedSandbox[, options])
Added in: v0.3.1
contextifiedSandbox
options
filename
lineOffset
columnOffset
displayErrors
timeout
breakOnSigint: if true, the execution will be terminated when SIGINT (Ctrl+C) is received. Existing handlers for the event that have been attached via process.on(“SIGINT”) will be disabled during script execution, but will continue to work after that. If execution is terminated, an Error will be thrown.
contextifiedSandbox
options
filename
lineOffset
columnOffset
displayErrors
timeout
breakOnSigint: 如果為真,當接收到(Ctrl+C)時,執行會被中斷。在腳本執行期間,連接到process.on(“SIGINT”)上存在的事件處理程序將會被禁用,但是在這之后腳本會繼續工作。如果執行被中斷了,就會拋出一個錯誤。
Runs the compiled code contained by the vm.Script object within the given contextifiedSandbox and returns the result. Running code does not have access to local scope.
The following example compiles code that increments a global variable, sets the value of another global variable, then execute the code multiple times. The globals are contained in the sandbox object.
運行編譯后的在給定的沙箱上下文中被vm.Script對象包含的代碼,然后返回其結果。運行的代碼無法獲取本地變量。
下面的例子編譯代碼,增加一個全局變量,設置其它全局變量的值,然后多次執行該代碼。全局變量被包含在一個沙盒對象中。
const util = require('util'); const vm = require('vm'); const sandbox = { animal: 'cat', count: 2 }; const script = new vm.Script('count += 1; name= "kitty"'); const context = new vm.createContext(sandbox); for(var i = 0; i < 10; i++) { script.runInContext(context); } console.log(util.inspect(sandbox)); //輸出 { animal: 'cat', count: 12, name: 'kitty' }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
script.runInNewContext([sandbox][, options])
Added in: v0.3.1
sandbox
options
filename
lineOffset
columnOffset
displayErrors
timeout
sandbox
options
filename
lineOffset
columnOffset
displayErrors
timeout
First contextifies the given sandbox, runs the compiled code contained by the vm.Script object within the created sandbox, and returns the result. Running code does not have access to local scope.
The following example compiles code that sets a global variable, then executes the code multiple times in different contexts. The globals are set on and contained within each individual sandbox.
首先將給定的沙盤上下文化,在創建的沙盤中運行被vm.Script對象編譯好的代碼,然后返回其結果。運行的代碼無法訪問本地范圍的對象。
下面的例子編譯代碼,然后設置一個全局變量,然后在不同的上下文中多次執行該代碼。全局變量被設置并包含在每一個單獨的沙盤中。
const util = require('util'); const vm = require('vm'); const script = new vm.Script('globalVar = "set"'); const sandboxes = [{}, {}, {}]; sandboxes.forEach((sandbox) => { script.runInNewContext(sandbox); }); console.log(util.inspect(sandboxes)); //輸出 [{ globalVar: 'set' }, { globalVar: 'set' }, { globalVar: 'set' }]
1
2
3
4
5
6
7
8
9
10
11
12
script.runInThisContext([options])
Added in: v0.3.1
- options
- filename
- lineOffset
- columnOffset
- displayErrors
- timeout
options
filename
lineOffset
columnOffset
displayErrors
timeout
Runs the compiled code contained by the vm.Script within the context of the current global object. Running code does not have access to local scope, but does have access to the current global object.
The following example compiles code that increments a global variable then executes that code multiple times:
運行被vm.Script包含的含有當前全局對象上下文的編譯好的代碼。運行的代碼不能訪問本地變量,但是可以訪問當前的全局對象。
下面的例子編譯增加一個全局變量的代碼,然后多次執行那個代碼。
const vm = require('vm'); //是否有加global都是全局變量 global.globalVar = 0; myVar = 0; const script = new vm.Script('globalVar += 1; myVar += 1', {filename: 'myfile.vm'}); for(var i = 0; i < 1000; i++) { script.runInThisContext(); } console.log(globalVar); // 1000 console.log(myVar); // 1000
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
vm.createContext([sandbox])
Added in: v0.3.1
sandbox
If given a sandbox object, the vm.createContext() method will prepare that sandbox so that it can be used in calls to vm.runInContext() or script.runInContext(). Inside such scripts, the sandbox object will be the global object, retaining all of its existing properties but also having the built-in objects and functions any standard global object has. Outside of scripts run by the vm module, sandbox will remain unchanged.
If sandbox is omitted (or passed explicitly as undefined), a new, empty contextified sandbox object will be returned.
The vm.createContext() method is primarily useful for creating a single sandbox that can be used to run multiple scripts. For instance, if emulating a web browser, the method can be used to create a single sandbox representing a window’s global object, then run all 亚洲真人日本在线| 伊人婷婷综合缴情亚洲五月| 亚洲av日韩综合一区在线观看| 亚洲七七久久精品中文国产| 国产精品亚洲一区二区在线观看| 亚洲女子高潮不断爆白浆| 亚洲熟妇AV乱码在线观看| 美女视频黄免费亚洲| 亚洲综合在线一区二区三区| 天堂亚洲国产中文在线| 亚洲一线产品二线产品| 亚洲丰满熟女一区二区哦| 亚洲а∨精品天堂在线| 偷自拍亚洲视频在线观看99| 亚洲国产a级视频| 久久久精品国产亚洲成人满18免费网站| 风间由美在线亚洲一区| 国产亚洲精品美女久久久久久下载| 男人的天堂亚洲一区二区三区 | 亚洲国产综合精品中文第一| 狠狠色伊人亚洲综合网站色| 亚洲人片在线观看天堂无码| 亚洲AV永久无码天堂影院| 无码专区一va亚洲v专区在线 | 久久丫精品国产亚洲av不卡| 亚洲毛片无码专区亚洲乱| 亚洲人成在线精品| 亚洲日韩国产二区无码| 国产偷国产偷亚洲高清在线| 亚洲人成人网站在线观看| 亚洲精品国产精品乱码不99| 亚洲最新永久在线观看| 亚洲人成免费网站| 国产偷国产偷亚洲清高APP| 亚洲日本在线观看视频| 亚洲Av永久无码精品三区在线| 久久精品国产亚洲精品2020| 亚洲一级免费视频| 亚洲av无码日韩av无码网站冲| 亚洲精品天堂成人片?V在线播放| 亚洲成AV人片在WWW色猫咪|